Project management
Now that you know how to program in pseudo-code, the aim is to show you how to adapt your pseudo-code programs so that they become real Python programs.
- First, we'll compare the first two programs in the pseudo-code chapter with their Python translations;
- Secondly, we'll list everything that's different in Python compared with pseudo-code.
By the end of this chapter, learners will have acquired a basic understanding of Python syntax, enabling them to create their first programs.
Comparing Python and pseudo-codeWe'll compare the first two pseudo-code programs with their Python translations. This will prepare you for Python syntax.
Pseudo-code exercise 1
Statement #1: The program must read numbers from the keyboard until it finds the value 0 (zero). The program must then indicate at which position this 0 appeared in the list of numbers read. A preliminary analysis is essential (in particular to identify the "trick(s)" required).
Here's a solution in pseudo-code.
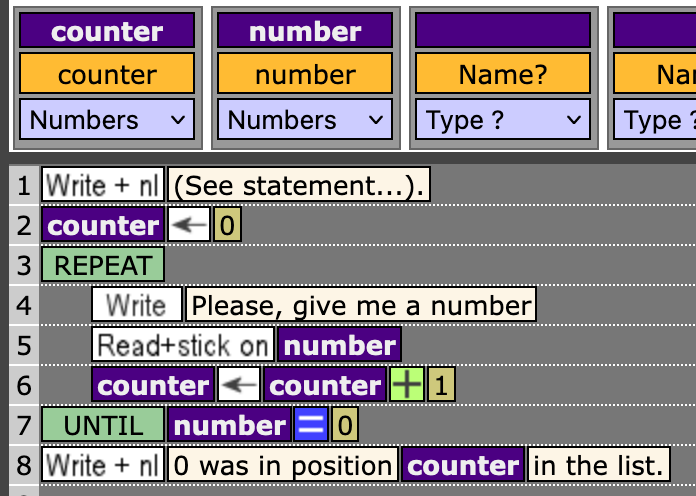
Here's the solution translated into Python.
On the left is the Python program itself. On the right is an example of how to run the program.

Full details of the translation can be found below.
Pseudo-code exercice 2
Statement #2: the program must read a maximum of 3 words, stopping reading as soon as it finds the word "stop". The program must then indicate whether the word "stop" was among the words read.
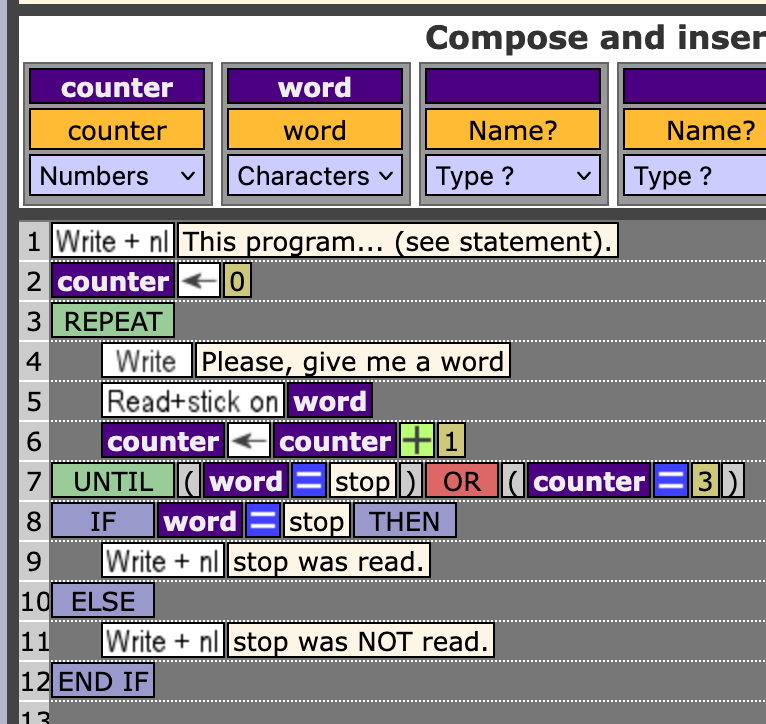
Here's the solution translated into Python.
On the left is the Python program itself. On the right is two examples of how to run the program.
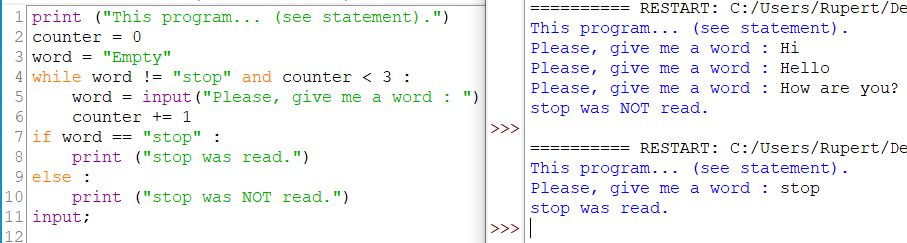
Full details of the translation can be found below.
Syntax in Python
The layout of Python programs or scripts requires great rigor, care and a keen sense of observation.
The indentations (indentation of text in relation to the margin) observed in virtual robots and pseudo-code will be of capital importance in program operation. Pay close attention to the positioning of these indentations to the nearest character. Here are the reasons why, compared with pseudo-code.
: The
and the
don't exist in Python
2 if verification :
3 instruction_2
4 instruction_3
5 instruction_4
6
7 instruction_5
The if in line 2 followed by the : (which replaces the then) will apply to instructions 2, 3 and 4. The end if on line 6 will not exist. It's the indentation return (the return to the margin) on line 7 that will stop the instructions covered by the if. The instruction_5 on line 7 is therefore not part of the if.
The
doesn't exist in Python
This is why de Morgan's theorem is so important. The human brain is more accustomed to thinking "right side up". The Repeat structure is therefore more natural than the While.
The good idea, to make things easier for yourself, is to think of the loop as a Repeat and create the stop condition of the Until as required. You can then use de Morgan's theorem to invert this condition and place it in the Until.
: The
and the
don't exist in Python
2 while verification :
3 instruction_2
4 instruction_3
5 instruction_4
6
7 instruction_5
The while in line 2 followed by : (which replaces the do) will cover instructions 2, 3 and 4. The end while on line 6 will not exist. It's the indentation return (the return to the margin) on line 7 that will stop the instructions covered by the while. The instruction_5 on line 7 is therefore not part of the while.
Data typesThe difference between a number and text: it's essential to be able to differentiate between the two.
123 is a number, and "123" or '123' are the sequences of characters one, two and three. A number represents a value that can be calculated with. It will be impossible to perform calculations with text. With text, on the other hand, you can isolate certain parts of a string, or a particular character for analysis, etc. Each type (number or character) is associated with specific processes that are not interchangeable.
Numbers
Numbers start with a digit, a dot (for decimal numbers) or a hyphen (for negative numbers). Any digit surrounded by quotation marks or apostrophes is a character, not a number. The dot determines the decimal part (e.g. 123.45 or .067). No comma can appear in a number.
There are three types of numbers: integers (integer), decimals (float) and complexes (rarely used).
The text
Quotation marks or apostrophes? Text is surrounded by quotation marks or apostrophes, but which to choose?
If you use a lot of quotation marks in your text, delimit it with apostrophes. If you use a lot of apostrophes, delimit your text with quotation marks.
If you use a mixture of both, here's how to solve the problem:
- If your text is delimited by apostrophes and an apostrophe needs to be displayed, use a \ (backslash) character in front of the apostrophe. Example: 'Hello, what\'s your first name?'
- If your text is delimited by quotation marks and a quotation mark is to be displayed, use \ (backslash) before the quotation mark. Example: "Is \"a\" the first letter of the alphabet?"
Booleans
We already know that there are two types of Boolean: true and false. In Python, they are written as : True and False with capital first letter and without quotation marks or apostrophes.
VariablesSyntax
In Python, you can create your own variable names, and it's a good idea to choose variable names that "mean something" and relate to their contents. This will make your programs easier to read and maintain.
However, you cannot use a reserved word such as "while", "range",... as a variable name, as these are part of the language. Apart from that, anything is possible, provided you observe the following rules:
- Variable names may contain letters, numbers and underscores ( _ ), but may not begin with a number;
- Variable names are case-sensitive, counter is different from Counter;
- Avoid accented and special characters (cedillas, etc.);
- The name of a variable cannot, of course, be enclosed in quotation marks or apostrophes, otherwise it becomes a character string and loses its variable status;
- Ideally, in Python, only lower-case letters should be used, possibly separating compound names with an underscore. For example, my_counter.
Declaration, creation
To declare or create a variable, simply assign (fill) it with its first value (initialize). The first use of a variable can take place anywhere in a program, except in a while condition where the variable is read. The variable must therefore be assigned at least once before this loop, but with a value that allows entry into the loop, otherwise the loop will be useless.
Assignment
In pseudo-code, we use the sign to assign (fill) variables. Of course, this acronym does not exist in Python. We use the equal sign ( = ) to make this assignment: the contents to the right of the = sign will be assigned to the variable whose name appears to the left of the = sign. Example: counter = 0 will assign the variable counter with the value 0. Consequently, the operator used to check equality in tests will be == to distinguish it from assignment.
Variable type
In Python, variable typing is dynamic. The variable will automatically take the type of the value you assign to it. Be careful when performing calculations: +, *... signs will have different effects and sometimes generate errors if the variable is recognized as a string. The use of mathematical functions such as round... allows you to force the variable type to numeric.
Display on screen and read from keyboard"print" will display its contents on the screen. print ("Hello") will display Hello on the screen. It's possible to display different things in succession, separated by commas between parentheses. Example: print ("The word", word, "was in position", counter, "in the list."). Note: Python will insert a space between each displayed element.
Two "prints" in a row will introduce a line break between them. This can be avoided by adding
, end = " ") at the end of the first "print". For example: print ("Your result is", end = " "). The content of the following print will be displayed on the same line following the display "Your result is ".
input
"input" is used to read data from the keyboard. For example: word = input ("Give me a word:"). The computer will display "Give me a word: ". The data then read from the keyboard will be assigned to the variable word.
Please note: any reading from the keyboard is done spontaneously in the form of characters. If you want to read an integer variable, for example, you need to add the int(...) function, which will instantly transform the reading into an integer. Example: my_integer = int (input ("Give me an integer:")). Don't forget the two closing parentheses. Use float to convert to decimal.
Operators in PythonThere are three types of operator in Python:
Arithmetic operators
+ (addition), - (subtraction), * (multiplication), / (division), ** (exponent), % (modulo, remainder of integer division) and // (integer division).
Comparison operators
== (equal to), != (not equal to), < (less than), > (greater than), <= (less than or equal to) and >= (greater than or equal to).
Boolean operators
or, and, not.
Control structures in PythonThere are three essential control structures in Python: the if-else conditional, the while loop and the for loop.
The if-else conditional (else is optional)
(1) instruction_1
(2) if condition:
(3) instruction(if)
(4) instruction(if)
(5) else:
(6) instruction(else)
(7) instruction(else)
(8) instruction_2
In Python, there's no such thing as "THEN" and "END IF" in pseudo-code: everything is based on layout, thanks to indentation (the indentation of instructions in relation to the margin).
The ":" in lines 2 and 5 introduce the contents of the if and else statements, which are identified by their indentation. Lines 3 and 4 will be executed if the condition is true, lines 6 and 7 if not. Lines 1 and 8 are executed in all cases. Note: the line numbers are there to help you understand, they don't have to be typed in with your program.
We've already mentioned that IF cascades are frequent and important to master. Here's how they look in Python, thanks to the reserved word elif, a contraction of the words else-if:
instruction_1
if condition:
instruction(if)
elif condition:
instruction(else)
else:
instruction(else-else)
instruction_2
The while loop
This loop is similar to the WHILE of pseudo-code, but the DO and END WHILE will not exist. As with the "if" loop, it is the layout that informs the computer of the instructions included in the loop. The while loop takes the following form:
(1) instruction_1
(2) while condition:
(3) instruction(while)
(4) instruction(xhile)
(5) instruction_2
Lines 3 and 4 are repeated as long as the condition is true. As soon as the condition is false, execution proceeds to line 5.
The for loop
Like the following loop, the while loop can be stopped by a break instruction when a condition is met.
(1) instruction_1
(2) for i in range(9):
(3) instruction(for)
(4) print(i)
(5) instruction_2
The loop will run 9 times, displaying values i from 0 to 8.
The value 9 in range could be replaced by the name of an integer variable. You can also choose the limits within which the counter will operate, for example:
for j in range(5, 15):
print(j)
The loop will display the value 5 on the first turn and stop before 15, so the last value displayed will be 14. Get used to this kind of thing.
We can also ask to spell out a string with the following instruction:
for x in "journal":
print(x)
The program will display all the letters in the word "journal" in columns, as the "j" is in position 0, the "o" in position 1, etc. This principle of always starting at 0 must be well integrated into coding.
An essential tool: listsA good command of lists will greatly facilitate coding in Python, as they are an exceptional tool with a large number of processing functions.
Declaration, creation
without_nothing = [ ] declares the without_nothing list empty, containing no elements.
tens = [10, 20, 30, 40, 50, 60, 70, 80, 90] declares the tens list containing integers from 10 to 90.
friends = ["Louisa", "John", "Salif"] declares the friends list containing 3 first names.
Methods
Lists are associated with a series of methods that make processing very easy. The syntax is list_name.method(...). Here are a few methods and their uses:
- - append(...) adds element ... to the end of the list.
- - clear( ) clears the list of all its contents.
- - copy() makes a copy of the list.
- - count(...) counts the number of times element ... appears in the list.
- - remove(...) removes element ... from the list.
- - reverse( ) reverses the order of elements in the list.
- - sort( ) sorts the list in ascending order.
Other methods exist and are worth examining. We'll look at these in greater depth in a next chapter.
CommentsComments are not executed by the computer, but simply indicate certain elements in the code text. It is essential to document these programs or scripts so that you can reread them and understand how they work. Here are two examples of comments:
- - # This instruction... : allows you to introduce "one-line" comments in scripts;
- - """ or ''' (3 quotation marks or 3 apostrophes) are used to introduce multi-line comments into scripts. The comment must end with the same character sequence.
If you get hooked on Python, you'll find a host of functions and libraries in every field that would be impossible to detail here without making it a website in its own right.
Once you've got the hang of it, use the Internet or recommended books to deepen your knowledge of the language and find functions that will make your work easier.
We wish you all the best in your exploration of Python.
Good luck and success.