This chapter is quite long – I suggest that you follow along with pen and paper and create a « report » of what follows that you can refer to as you continue with your learning.
What is JavaScript (« JS » for short)?
JavaScript is a programming language that lets you write a « program » or « script » to control the behavior of your computer. JS is made up of instructions that trigger specific, basic behaviors in the machine. For example, the instruction « 12 + 4 » asks it to carry out this mathematical operation, and the result returned is « 16 ».
Difference between « instruction » and « function »
As we just saw, an instruction triggers a behavior in the computer that is « elementary ». More complex behaviors require a series of instructions. By combining sets of instructions into specific structures we are able to create a « function ».
The advantage of a function is that it can be defined once and later « called » (executed) as many times as you wish. This lets you save a lot of space in your script and makes the script easier to read. The full series of instructions is then represented by a label: this is the « function name ».
Additionally, some functions are called by putting one or more « parameters » or « arguments » in parentheses. This is information that will be used to give instructions to the function. For example, if a function is called « mySquare » and calculates the square of a number (the number multiplied by itself), then when the function is called, the number to be squared must be provided. So for example, for the number 8, this becomes : mySquare (8); and the function will return « 64 ».
« Reserved Words » and « My Words »
The computer must be able to distinguish between words used for its own instructions and words you choose for your own use (for example, the name of a function you write yourself). So there some words you can’t use because they are reserved for use as your computer’s own commands. Here is an example :
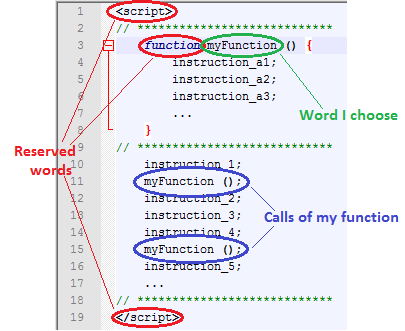
Unpacking the Information Contained in this Example
This short example of script provides a lot of information on how to program in JavaScript. Let’s take a closer look.
1. Reserved Words, Statements,...
This script distinguishes between reserved words (circled in red) and words chosen to name my new function (circled in green).
As you can see, the definition of my new function is introduced (« declared ») by the reserved word « function » and is followed by a name I chose, along with its accompanying instructions, which are surrounded by the curly braces « { » and « } ».
Later in the program (script), my function can be called by simply entering its name.
We’ll talk more about parentheses later.
2. Organizing the Script (Layout and Syntax)
To simplify, instructions are separated from one another using semicolons (;) – this is the case in most computer languages. Refer to the Web for the finer details of this.
To facilitate reading, indents (wider margins for select lines of text) are used for some structures of the language. We will return to this point later.
3. Upper & Lower Case
As you can see, the entire program is written in lowercase – this is standard in JS. There is, however, a capital letter in « myFunction ». In JS, when combining words into one, it is customary for each new word to start with a capital letter to make the new word easier to read.
Note: JS distinguishes between uppercase and lowercase letters. This means that « myFunction » is different from « myfunction ». So you need to be careful – this is a common source of errors.
4. Characters Valid for Use in « My Words »
Without going into too much detail, the basic guidelines for character use are:
- • Use lowercase and uppercase letters, numbers, underscores (_) and dollar signs ($);
- • Always start « your word » with a letter, underscore (_) or dollar sign ($), but never with a number;
- • No other characters, such as @, -, ^ or blank spaces are allowed.
5. Numbers, Strings and Booleans
Note: Strings are different from « my words ». « My words » are used to name functions and variables, while strings are sentence fragments entered on the keyboard which are then manipulated in order to be read on the screen.
Strings must be set out by quotation marks (") or apostrophes ('). This is not the case for numbers, Booleans or « my words ». So there is a difference between 15 , "15" and '15'. The first is recognized as a number and the next two are recognized as sequences of characters.
So 15 + 15 will return the result 30, and "15" + '15' will return the result 1515.
Character strings can contain any character on the keyboard except for delimiting characters. A string delimited with quotation marks (") cannot contain quotation marks unless they are preceded by a backslash (\), which is known as an escape character. The same is true if you choose the apostrophe (') as your delimiting character: if you wish to use an apostrophe (') in a string, it must also be preceded by a backslash (\).
Numbers can be whole numbers (integers) or real numbers (containing decimals). The fractional part is set off with a period, as for example, 9.81. The accuracy is 252, i.e., greater than 1015 (a 1 followed by 15 zeros). Within this range, all operations will return an exact result, with the exception of some decimals from which a certain number of decimals may be cut off.
Booleans are special data types that can only have two values: « true » or « false », without quotation marks (") or apostrophes (') as delimiters.
6. Notes
Comments can be included in scripts. These comments are instructions intended to help understand certain areas of a script that may be difficult to interpret.
A one-line comment starts with // and continues until the end of the line.
A multi-line comment begins with /* and ends with */.
That’s it!
I think you now have the background needed to begin the exercises. Now it’s your turn...!!!