Objectives
- In this chapter you will gain a deeper understanding of conditionals and their syntax;
- You will encounter nested conditionals and learn how to use them.
Nested conditionals
In the last chapter we saw how a simple conditional structure is used, and how it lets you make the choice between two possibilities: heads or tails.
But what should you do when there are more than two possibilities? How do you determine what actions to take based on the outcome of tests?
Your Challenge, Your Task
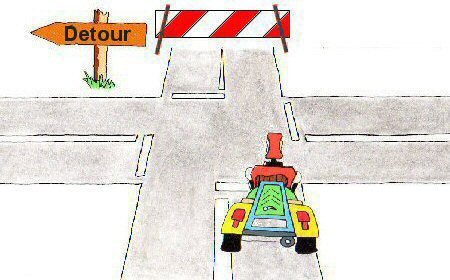
Write your code so that the robot goes the right way.
The robot is able to drive its vehicle and react to traffic information such as detours.
The driver robot usually goes straight ahead, but if there is a left or right detour at the intersection, it must turn left or right.
Nested Conditional Control Structures
We have seen that in simple conditional structures we can create a test that turns out either true or false, i.e., one of two possibilities. But in this situation, we must decide between three possibilities: going left, right or straight ahead.
Let's start with the case of a detour to the left:
- if (the result of the test « left detour » is true)
- turn left
- else
- it still isn't clear: go right or go straight?
We see that an unanswered question remains in the « otherwise » since there are still two directions to decide between. This can be handled by using another « if-statement ».
So we end up with two conditional structures which are nested as follows:
- if (the result of the test « left detour » is true)
- turn left
- else if (the result of the test « right detour » is true)
- turn right
- else
- go straight ahead
So we were able to guide the robot in one of 3 possible directions using a « cascading if-statement » with 2 nested if-statements.
So how does that work in JavaScript?
In JavaScript, the syntax is as follows:
- if (test 1)
- action to carry out if test 1 returns the result true;
- else if (test 2)
- action to carry out if test 2 returns the result true;
- else
- action to carry out if (test 1 and) test 2 return the result false;
Be aware that the « else of the else » leads to a « place » where neither Test 1 or Test 2 resulted in true. The advantage of « if cascades » is that at every level, all the true results of the previous tests are excluded. This is a very important concept to grasp.
Please note that indenting the instructions contained in the conditional has no effect on the execution of the code, but it is a good habit to get into to make your scripts easier to read.
Available functions
The functions available for this exercise are as follows:
- The action function drive (); the robot drives straight up to the next crossroads;
- The action function go ('direction'); where 'direction' = 'left', 'right' or 'straightAhead';
- The test function detourLeft () which returns true or false;
- The test function detourRight () which returns true or false.
Now it’s your turn to apply everything you’ve just read above – good luck!