Objectives
- This chapter will help you to deepen your understanding of nested conditionals.
Nested conditionals (continuation)
In the previous chapter we saw that by using two nested conditional structures, a choice could be made from among three possibilities.
This is the same type of exercise, except that there are now 4 possibilities.
Your Challenge, Your Task
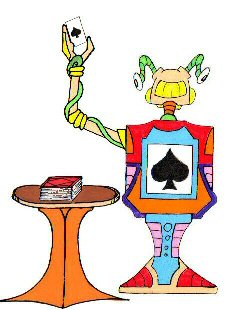
Write code to make the robot display the right card.
The robot is able to pick up a deck of cards, pick a card, and show the card. It can also tell what suit the card is in: hearts, diamonds, clubs or spades.
The robot must pick a single card from the deck and show what card it is.
Nested Conditional Control Structures
In the previous exercise, we saw how to decide between 3 possible actions on the basis of 2 tests.
This exercise is a bit more complicated, but not really different from the previous one: you must decide between 4 possible actions on the basis of 3 tests.
All you have to do is add one additional level to the nested conditionals introduced in the previous exercise.
In JavaScript, this looks like this:
- if (test 1)
- action to carry out if test 1 returns the result true;
- else if (test 2)
- action to carry out if test 2 returns the result true;
- else if (test 3)
- action to carry out if test 3 returns the result true;
- else
- action to carry out if test 3* returns the result false;
* Once again, pay close attention to the fact that, as in the previous exercise, in the « else of the else of the else » we arrive at a point at which neither Test 1, Test 2 or Test 3 returns a true result. The advantage of « if cascades » is that at each level, all the true results of previous tests are excluded. This is a very important concept to grasp.
Available functions
The functions available for this exercise are as follows:
- The action function pickCard ();, without an argument: the robot picks a card from the deck and shows it;
- The action function show ('?');, the robot shows which card it picked – the argument to be entered in '?' is either 'hearts', 'diamonds', 'clubs' or 'spades', depending on the card picked;
- The test function heartsCard (), which returns true or false;
- The test function diamondsCard (), which returns true or false;
- The test function clubsCard (), which returns true or false.
Now it’s your turn to apply everything you’ve just read above – good luck!