Objectives
- This chapter will help you to deepen your understanding of nested conditionals.
Nested conditionals (continuation)
In the previous chapter we saw that by using 3 nested conditional structures, we were able to make a selection from among 4 possibilities.
This is the same type of exercise, except that you will now have only 2 tests to use to choose between 4 possibilities.
Your Challenge, Your Task
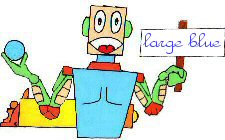
Write your code to make the robot show which ball it caught.
The robot can throw 4 balls in the air, then catch and show one ball. It can also check what kind of ball it is: small or not small, blue or not blue.
The robot must show whether it caught the small blue ball, the small yellow ball, the large blue ball or the large yellow ball.
Nested Conditional Control Structures
This exercise is a bit more complicated, but not terribly different from the previous one: 4 actions must be decided on based on 2 tests.
This is possible because each ball is defined by two characteristics: size and color.
You will have to organize the « if-cascades » in such a way as to arrive at the result. Unlike previous exercises, we are not giving you the solution, so you'll need to get a piece of paper and pencil and become the « architect » of your own if-cascade.
Available functions
The functions available for this exercise are as follows:
- The action function throw4Balls (); without an argument: the robot throws 4 balls in the air;
- The action function catch1Ball (); without an argument: the robot catches 1 of the 4 balls that fall;
- The action function show ('?'); the robot shows which ball was caught. The argument to be entered in '?' should be either 'largeBlue', 'largeYellow', 'smallBlue', or 'smallYellow' depending on which ball is caught;
- The test function smallBall () returns true or false;
- The test function blueBall () returns true or false;
Now it's your turn to apply everything you've just read above – good luck!