Objectives
- To understand how loops work;
- To correctly use loops in the following exercises.
Your Task, Your Challenge
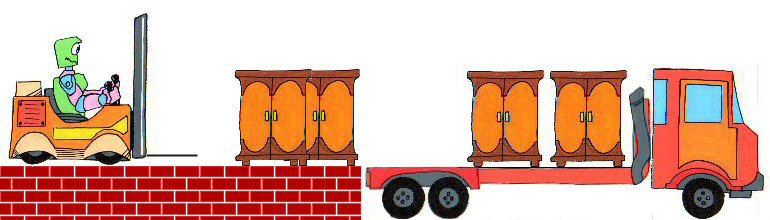
Write your code so that the robot loads the right amount onto trucks of various sizes.
Write your code so that the robot loads as many cabinets as possible onto each truck that arrives. Note that some trucks can carry more cabinets than others. Whatever size truck arrives, the robot must keep loading it until it is full.
There is one test for this:
- truckFull() checks whether the truck is fully loaded, no matter what its size.
Loops
The robot can only load one cabinet at a time. It must start over each time, doing the same action repeatedly. This is handled as follows:
- do
- load() a cabinet on the truck;
- comeBack() on the loading dock;
- while not truckFull();
Naturally this repetitive control structure exists in JS, and looks like this:
- do
- singleInstruction;
- while (condition);
The « do » accepts only one instruction. If you wish to include multiple instructions, you must combine them into a single block of code using the curly braces « { » and « } ».
The JS code then becomes:
- do {
- instruction_1;
- instruction_2;
- instruction_3;
- }
- while (condition);
It’s your turn!!!