Objectives
- Understanding that there are two types of loops;
- Recognizing which type of loop to use in various cases;
- Correctly using loops in the following exercises.
Your Task, Your Challenge
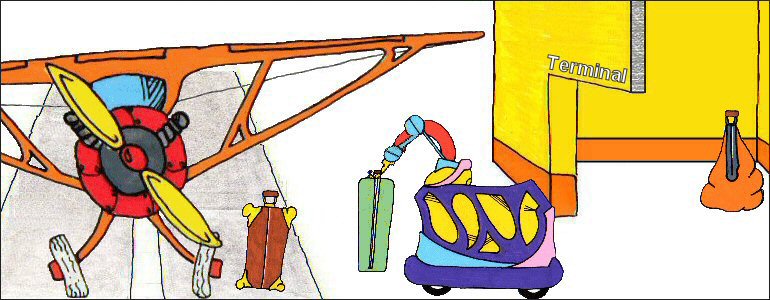
Write your code so that the robot brings all the luggage into the airport terminal.
You must write your code so that the robot brings all the luggage into the airport terminal. Note that sometimes there are no suitcases on board the plane. But regardless of how many suitcases have been transported, the robot must bring them all inside.
There is a test available to do this:
- luggageOnRunway() which checks whether there are any more suitcases on the runway.
Repetition statements
In the previous exercise (the cabinet-loading robot), the robot had at least one cabinet to load onto any truck. The structure « do ... while... » was applied to the situation:
- do
- load() a cabinet on the truck;
- comeBack() on the loading dock;
- while (not truckFull());
In this new case, however, it’s possible that there are no suitcases to transport. So we cannot do the action until testing whether there is at least one suitcase to be transported. It is therefore necessary to use a repetition statement « that tests before acting ». This can be structured in JS as follows:
- while (luggageOnRunway())
- do what is required to bring in the luggage;
This repetitive control structure exists in JS as follows:
- while (condition)
- singleInstruction;
Here « while » only accepts a single instruction. If you wish to include more statements, you must combine them into a single block of code using the curly braces « { » and « } ».
The JS code then becomes:
- while (condition) {
- instruction_1;
- instruction_2;
- instruction_3;
- }
It's your turn!!!
Good luck.